Let's add some data to your DBs.
Start phpMyAdmin on localhost
. Select the wombats table in the wombats DB.
Use the Insert tab, and fill in the fields for a new record, but leave the id MT (empty). Remember, we set the id field to auto increment. MySQL will set the value for us.
Save the record. You'll see something like:
Checkout the INSERT statement:
- INSERT INTO `wombats` (`wombat_id`, `name`, `weight`, `comments`)
- VALUES (NULL, 'Muddle', '27', 'A muddle headed wombat.');
This is SQL, a statement that will create a new record. It's just a string, that you could make yourself in code. You'll be doing that later.
Click the Create PHP code link. You'll see:
- $sql = "INSERT INTO `wombats` (`wombat_id`, `name`, `weight`, `comments`) VALUES (NULL, \'Muddle\', \'27\', \'A muddle headed wombat.\')";
Your code will look something like that.
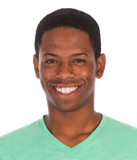
Ethan
What are the quotes and slashes and things for?
The whole string is inside double quotes: "INSERT INTO ... ";
. That's so PHP knows where the string starts and ends.
The `s are called back ticks, or back quotes. They let you put spaces into table and field names. For example, suppose we'd named the table cute wombats
. We'd put `cute wombats`
into the SQL, so the DBMS would know that we had a space in the table name.
However, do not put spaces in table or field names. Use an _, as in wombat_id
. Using spaces, while technically OK, is asking for trouble.
Since there aren't spaces in table or field names, the PHP could be...
- $sql = "INSERT INTO wombats (wombat_id, name, weight, ...
However, phpMyAdmin isn't smart enough to omit the back ticks.
It's the same with the \s. They don't need to be there in this case, so the code could have been:
- $sql = "INSERT INTO wombats (wombat_id, name, weight, comments) VALUES (NULL, 'Muddle', 27, 'A muddle headed wombat.')";
Notice that 27
doesn't need 's around it, since it's a number.
Copy the record to the server
Copy this SQL:
- INSERT INTO wombats (wombat_id, name, weight, comments) VALUES (NULL, 'Muddle', 27, 'A muddle headed wombat.')
If it's right, it should work when run on the server. Give it a try. In phpMyAdmin on the server, go to the SQL tab, and paste that code in.
Hit Go, and you should get:
BTW, notice how long it took: less than 1 millisecond. A millisecond is 1,000th of a second. A fast eye blink takes about 100 milliseconds. So MySQL could store about 100 records in the time it takes you to blink an eye.
More records
There are 17 more wombat records you can download. Grab the download, and import into both your databases, the localhost
one, and the Reclaim one.
What's next?
Let's see how you query the DB.