Emily's doggos
The select
tag
Here's a dropdown.
They're really called select widgets, but many people just call them dropdowns, since that's what they do.
Here's the HTML for that.
- <select>
- <option>Dogs</option>
- <option>are</option>
- <option>the</option>
- <option>best</option>
- </select>
Here's a more realistic example.
- <label>Please choose the best animal<br>
- <select name="best-animal">
- <option value="none">(Choose)</option>
- <option value="catto">Cat</option>
- <option value="doggo" selected>Dog</option>
- <option value="llamo">Llama</option>
- <option value="poly">Parrot</option>
- </select>
- </label>
(The (Choose) option is an instruction for users, and a placeholder for "nothing chosen yet.")
The widget's value in the POST
array will have the index best-animal
, since that's what the name
is.
The value
property gives the value of the POST
element.
- If the user chooses Cat,
$_POST['best-animal']
will becatto
. - If the user chooses Parrot,
$_POST['best-animal']
will bepoly
.
The option the user has chosen will have the selected
property in the HTML. That's why the dropdown showed Dog when you opened this page:
- <option value="doggo" selected>Dog</option>
Choosing multiple items
Here's the animal one again, but with some extra stuff.
All that's changed is the select
tag.
- <label>Please choose the best animal<br>
- <select name="best-animal" multiple size="5" >
- <option value="none">(Choose)</option>
- ...
multiple
means users can choose more than one option. Try it. Click on one, then Ctrl+click on another.
size
is the number of items that are shown. If size
is less than the number of items in the list, you get a scroll bar.
Multiple selection in POST
Here's that thing again.
So, a user chooses some values, and submits the form. The processing page gets values the user chose. How will the processing page know which values the user choose?
PHP has a little trick here. Put []
at the end of the name
property:
- <select name="best-animal[]" multiple size="5">
$_POST['best-animal']
will be an array, listing all the values the user chose.
Simple example
At the start of this section, we had two simple pages:
- A form with a widget, that passed data to...
- A page that showed the user's input.
Let's go back to that, without DBs, to see how the dropdown widget works. You can try it, and download the code.
Here's the HTML, same as before, but with []
added to name
.
- <select name="best-animal[]" multiple size="5">
- <option value="none">(Choose)</option>
- <option value="catto">Cat</option>
- <option value="doggo" selected>Dog</option>
- <option value="llamo">Llama</option>
- <option value="poly">Parrot</option>
- </select>
Let's see what different user inputs look like to the processing page, and what output we want. We have to allow for several different scenarios:
If user choice... | Then $_POST['best-animal'] is... | Desired output |
---|---|---|
User selected no options![]() |
Does not exist![]() |
![]() |
Selected only (Choose)![]() |
One element, with a value of none![]() |
![]() |
Selected one or more animals![]() |
Animals![]() |
![]() |
Selected one or more animals, and (Choose)![]() |
Animals, and none![]() |
![]() |
The table guides the code we should write.
PHP processing dropdowns
- <?php
- // Fill $message, and show it in the HTML.
- $message = '';
- if (!isset($_POST['best-animal'])) {
- // Nothing selected.
- $message = "You didn't choose any animals.\n";
- }
- if ($message == '') {
- // Test whether just the (Choose) option was chosen.
- $chosen = $_POST['best-animal'];
- if (count($chosen) == 1 && $chosen[0] == 'none') {
- $message = "You didn't choose any animals.\n";
- }
- }
- if ($message == '') {
- // User chose animals.
- $message = "<p>You chose:</p>\n<ul>\n";
- foreach ($chosen as $animal) {
- // Skip the none option.
- if ($animal != 'none') {
- $animal = ucfirst($animal);
- $message .= "<li>$animal</li>\n";
- }
- }
- $message .= "</ul>\n";
- }?>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <title>Best animals</title>
- <link rel="stylesheet" href="library/styles.css">
- </head>
- <body>
- <h1>Best animals</h1>
- <p><?php print $message; ?></p>
- </body>
- </html>
Lines 4-7 handle the nothing-chosen case, using isset()
to see if $_POST['best-animal']
exists. If it doesn't, the user didn't choose anything.
Lines 10-13 cover the situation where users selected only the (Choose) option, and none of the animals. It might not make sense for users to choose that, but the code still allows for it.
What does the count()
function in line 11 do?
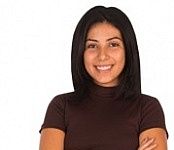
Adela
It tells you how many elements are in an array.
Aye!
Lines 18-24 process animal selections. Line 20 skips the (Choose) case, if it was selected.
Let's run through that last bit. Suppose I did this...
... and hit the Go button, send the data for processing.
This code...
- $chosen = $_POST['best-animal'];
... puts data from POST for the widget into $chosen
. What's in there? Here's what the debugger says:
$chosen
is an array, with two elements, each one the value
of the thing the user selected:
- <select name="best-animal[]" multiple size="5">
- <option value="none">(Choose)</option>
- <option value="catto">Cat</option>
- <option value="doggo" selected>Dog</option>
- <option value="llamo">Llama</option>
- <option value="poly">Parrot</option>
- </select>
So, let's loop over the array, and make HTML for each of the elements. We want this:
- <ul>
- <li>Doggo</li>
- <li>Poly</li>
- </ul>
Here's the code again:
- $message = "You choose:<br>\n<ul>\n";
- foreach ($chosen as $animal) {
- // Skip the none option.
- if ($animal != 'none') {
- $animal = ucfirst($animal);
- $message .= "<li>$animal</li>\n";
- }
- }
- $message .= "</ul>\n";
Line 1 makes the opening ul
. Line 9 closes the ul
. The code between them makes all the li
s inside the ul
.
Now, $chosen
has two elements, doggo
, and poly
. The first time through the loop, $animal
will be doggo
:
What does ucfirst
do?
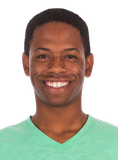
Ethan
Makes the first character of a string uppercase.
Right! It turn doggo
into Doggo
, better for output.
The second time through the loop...
...@$animal@ is poly
.
The HTML is accumulated in $message
. Not simply output it.
- <body>
- <h1>Best animals</h1>
- <p><?= $message ?></p>
- </body>
Exercise
Pokemon evolutions
Make a PHP app showing the evolutions for Pokemon the user selects. There's a form with a dropdown showing a few Pokemon, like this:
Click the button, and the user would see:
With this input...
... the user would see...
You can try my solution
Choose any Pokemon you want, at least four.
Submit your URL, and a zip of your files.
Up next
Let's grab options for dropdowns from the DB.