Matthew's Maggie
So far, you've gotten user data from the end of a URL. That's used a lot in real apps, to send data between pages. However, when people type data, you want to use forms.
You've seen lots of forms on the web. They have various types of fields, like text boxes.
Best animal
Checkboxes:
Are you funny?
Drop downs (selects):
... and other widget types. Yes, we'll call them widgets.
This section of the course is about using forms to capture data, update it, delete it, and report on it.
The form tag
Let's make a simple form like this:
It has one text widget, and one button. You can try it.
Here's the HTML
- <form method="post" action="check-simple-form-data.php">
- <p>
- <label>
- What's the best animal?<br>
- <input type="text" name="best-animal" size="20"><br>
- <small>Hint: woof!</small>
- </label>
- </p>
- <p>
- <button type="submit">Save</button>
- </p>
- </form>
You can try it.
Let's look inside the form tag first. This...
- <input type="text" name="best-animal" size="20">
... makes the text widget. It's about 20 characters wide. Its name is best-animal
; that will be important in a mo.
The widget is wrapped in a label
tag.
- <label>
- What's the best animal?<br>
- <input type="text" name="best-animal" size="20"><br>
- <small>Hint: woof!</small>
- </label>
Clicking on the label is the same as clicking on the widget. It puts the keyboard cursor in the widget. Try the app, and click on the label.
Here's the form code again.
- <form method="post" action="check-simple-form-data.php">
- <p>
- <label>
- What's the best animal?<br>
- <input type="text" name="best-animal" size="20"><br>
- <small>Hint: woof!</small>
- </label>
- </p>
- <p>
- <button type="submit">Save</button>
- </p>
- </form>
After the text widget, there's a button. The button's type
is submit
; that's important. Clicking a submit
button in a form will tell the form to send its data somewhere for processing.
Note
Click a submit
button to send a form's data somewhere.
The form
's action
gives the URL to send the data to. So, click the button, and the form will send its data to check-simple-form-data.php
.
The method
...
- <form method="post" action="check-simple-form-data.php">
...is either post
, or get
. get
attaches the form data to the end of the action
's URL, as we've been doing for a while. post
sends the data through HTTP headers, which is normally what you want, since the data is hidden from the user. (What is an HTTP header? We don't care. It works.)
Receiving form data
OK, so the data is sent to check-simple-form-data.php
. Here's code from that page.
- <?php
- // Get user data
- $bestAnimalInput = strtolower(trim($_POST['best-animal']));
- // Message to show.
- $message = '';
- // Class to apply to the message.
- $messageClass = '';
- if ($bestAnimalInput == 'dog' || $bestAnimalInput == 'dogs') {
- $message = 'Yes, the dog is the best animal.';
- $messageClass = 'right';
- }
- else {
- $message = "No, $bestAnimalInput is not the best animal.";
- $messageClass = 'wrong';
- }
- ?><!DOCTYPE html>
- ...
- <p class="<?php print $messageClass; ?>"><?php print $message; ?></p>
The important bit is line 3. Just as there's a $_GET
array, there's a $_POST
array. This program grabs the user's data with $_POST['best-animal']
.
Why does line 3 use best-animal
?
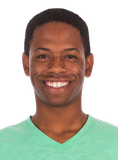
Ethan
Because that's the name of the text widget.
- <input type="text" name="best-animal" size="20">
Right! You need to add a name
property to every widget, if you want to be able to get the user's data.
What happens to the data?
- <?php
- // Get user data
- $bestAnimalInput = strtolower(trim($_POST['best-animal']));
- // Message to show.
- $message = '';
- // Class to apply to the message.
- $messageClass = '';
- if ($bestAnimalInput == 'dog' || $bestAnimalInput == 'dogs') {
- $message = 'Yes, the dog is the best animal.';
- $messageClass = 'right';
- }
- else {
- $message = "No, $bestAnimalInput is not the best animal.";
- $messageClass = 'wrong';
- }
- ?><!DOCTYPE html>
- ...
- <p class="<?= $messageClass ?>"><?= $message ?></p>
Once you've put the POST
data into a variable, it's like any other variable. This code...
- strtolower(trim($_POST['best-animal']))
...strips out extra spaces from the user's input, and converts it to lowercase, to make testing the value easier.
The if
checks whether the user got the answer right. $message
is the text that will be shown to the user. $messageClass
is a CSS class that will be applied to the message:
- <p class="<?= $messageClass ?>"><?= $message ?></p>
Split input and processing
Note that we have two pages here.
- A page with a form, handling user input. It sends data to...
- A second page that shows the data.
We could combine input and processing on one page, but the code would be more complex. Let's use the split-input-and-processing pattern from now on.
Separate input from processing
Put input and validation in one program. Put processing code in another. Use the session to send data from one to the other.
Exercise
Pokemon petting zoo tickets
Compute the price of tickets to a Pokemon petting zoo. Make a form like this:
Users enter the number of adults and kids in their group. Assume users always input numbers that are zero or more. For example:
Show the price, where adult tickets are $15, and kid tickets are $8.
If adults is zero, and kids is more than zero, show an error message.
Submit your solution URL, and a zip of your files. The usual coding standards apply.
Up next
Let's do some validation.