Where are we?
The code to check permission, and change menus, relies on two functions:
isLoggedIn()
: returns true if the user is logged inhasRole($roleToCheck)
: returns true if the user has the role
With these two functions, we can make a simple user login system.
The two functions use data from the session to do their thing.
isLoggedIn()
isLoggedIn()
relies on $_SESSION['logged_in']
, a boolean:
- /**
- * Is the current user logged in?
- * @return bool True if logged in, else false.
- */
- function isLoggedIn() {
- $loggedIn = false;
- if (isset($_SESSION['logged_in'])) {
- if ($_SESSION['logged_in']) {
- $loggedIn = true;
- }
- }
- return $loggedIn;
- }
The code uses the flag pattern.
Use a variable as a flag. Set the flag if any of a number of things happens. After, check the flag to see if any of those things happened.
hasRole($roleToCheck)
hasRole($roleToCheck)
relies on $_SESSION['user_roles']
to do its work.
- /**
- * Does the current user have the given role?
- * @param string $roleName Role name, using consts above.
- * @return bool True if has role, else false.
- */
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
Users table
We have a DB table with user names, password hashes, and roles.
What login should do
Here's the basic logic for login:
- Show a login form.
- When the user types in a username and password:
- Compare the username and password hash to the DB
- If they match:
- Put data into the session
Code on pages we want to protect will check the data in the session.
How to implement logout? The easiest way is to destroy the session.
The form
Here's what the form should look like:
It's a Bootstrap form, of course.
Earlier, you learned how to have form input and validation on the same page. Let's do that again.
The code
Here it is.
- <?php
- require_once 'library/useful-stuff.php';
- // Already logged in?
- if (isLoggedIn()) {
- header('Location: index.php');
- exit();
- }
- $errorMessage = '';
- // Load default values for widgets.
- $username = '';
- $password = '';
- // Is there post data?
- if ($_POST) {
- // User filled in data and sent it to this page.
- // Grab the data sent.
- $username = getParamFromPost('username');
- $password = getParamFromPost('password');
- // Is it valid?
- $success = logIn($username, $password);
- if ($success) {
- // Back to home page.
- header('Location: index.php');
- exit();
- }
- // Show an error message.
- $errorMessage = 'Sorry, login failed.';
- }
- ?><!doctype html>
- <html lang="en">
- ...
- <h1>Login</h1>
- <?php
- // If there's an error message, show it.
- if ($errorMessage != '') {
- print "<div class='alert alert-danger' role='alert'>$errorMessage</div>";
- }
- ?>
- <form method="post">
- <div class="mb-3">
- <label for="username" class="form-label">User name</label>
- <input type="text" class="form-control" name="username"
- value="<?= $username ?>"
- >
- </div>
- <div class="mb-3">
- <label for="password" class="form-label">Password</label>
- <input type="password" class="form-control" name="password"
- value="<?= $password ?>"
- >
- </div>
- <button type="submit" class="btn btn-primary">Login</button>
- </form>
- ...
Let's check it out. If the user is already logged in, then they don't need to log in again, so we can leave.
- if (isLoggedIn()) {
- header('Location: index.php');
- exit();
- }
Notice how easy to understand the code is. The function isLoggedIn()
is true when... well, when the user is logged in. Set up and name your functions well, and your code is easy to understand, easy to debug, and easy to modify. That's three easys!
Init $errorMessage
, and variables representing values for the widgets. Part of the same-page validation.
- $errorMessage = '';
- // Load default values for widgets.
- $username = '';
- $password = '';
If there's POST data, put it into the variables we just made, and check it.
- if ($_POST) {
- // User filled in data and sent it to this page.
- // Grab the data sent.
- $username = getParamFromPost('username');
- $password = getParamFromPost('password');
- // Is it valid?
- $success = logIn($username, $password);
- if ($success) {
- // Back to home page.
- header('Location: index.php');
- exit();
- }
- // Show an error message.
- $errorMessage = 'Sorry, login failed.';
- }
Line 19 is:
- $success = logIn($username, $password);
logIn()
is a new function, in the library. We send it the user name and password from the form. It returns either true, or false. It also stores the right data in the session.
If logIn()
returns true, we jump to the home page. If not, the program continues, and shows the form again, but with an error message above it:
- <h1>Login</h1>
- <?php
- // If there's an error message, show it.
- if ($errorMessage != '') {
- print "<div class='alert alert-danger' role='alert'>$errorMessage</div>";
- }
- ?>
- <form method="post">
- ...
- </form>
logIn()
Here's some pseudocode for the function.
- If the username or password are missing:
- Return false (login failed)
- Hash the password
- Check the DB for a user record with the username and hashed password
- If it isn't there:
- Return false (login failed)
- // If get to here, username and password are OK.
- Put user data into the session
- Return true (login OK)
Here's the code, from the library.
- /**
- * @param string $userName Username.
- * @param string $password Password.
- * @return bool True if login succeeded, else false.
- */
- function logIn($userName, $password) {
- global $dbConnection;
- $userName = strtolower(trim($userName));
- $password = trim($password);
- // Exit if either is MT or null.
- if ($userName == '' || is_null($userName)
- || $password == '' || is_null($password) ) {
- return false;
- }
- $hashedPassword = md5($password);
- $sql = 'select * from users where name=:username and password_hash=:pw_hash;';
- /** @var PDO $dbConnection */
- $stmnt = $dbConnection->prepare($sql);
- $isQueryWorked = $stmnt->execute([
- ':username' => $userName,
- ':pw_hash' => $hashedPassword
- ]);
- if (! $isQueryWorked ) {
- // Query did not work.
- return false;
- }
- if ($stmnt->rowCount() != 1) {
- // Row not found.
- return false;
- }
- $userEntity = $stmnt->fetch();
- // Store data in session.
- $_SESSION['username'] = $userEntity['name'];
- $_SESSION['user_roles'] = explode(',', $userEntity['roles']);
- $_SESSION['logged_in'] = true;
- return true;
- }
First, it makes sure both the username and password are given.
- $userName = strtolower(trim($userName));
- $password = trim($password);
- // Exit if either is MT or null.
- if ($userName == '' || is_null($userName)
- || $password == '' || is_null($password) ) {
- return false;
- }
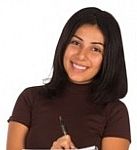
Adela
Oh, that's cool. The username and password are both trimmed, so if someone hits the spacebar after typing them in, it won't matter.
The username isn't case-sensitive, but the password is.
Right! Another reason there might be an extra space, is if the user copy-and-pasted, and didn't get the text selection quite right. It's easy to accidentally include a space.
The first validation (lines 11 to 14) is both fields are required. I could have made the test simpler, but I tested for both MT and null, just in case.
We're up to:
- If the username or password are missing:
- Return false (login failed)
- UP TO HERE
- Hash the password
- Check the DB for a user record with the username and hashed password
- If it isn't there:
- Return false (login failed)
- // If get to here, username and password are OK.
- Put user data into the session
- Return true (login OK)
Now, let's check to see if the username and password are in the DB. Actually, we know the password is not in the DB, because we don't store passwords. We store hashes, instead. So:
- $hashedPassword = md5($password);
- $sql = 'select * from users where name=:username and password_hash=:pw_hash;';
- /** @var PDO $dbConnection */
- $stmnt = $dbConnection->prepare($sql);
- $isQueryWorked = $stmnt->execute([
- ':username' => $userName,
- ':pw_hash' => $hashedPassword
- ]);
- if (! $isQueryWorked ) {
- // Query did not work.
- return false;
- }
- if ($stmnt->rowCount() != 1) {
- // Row not found.
- return false;
- }
- $userEntity = $stmnt->fetch();
If there isn't a row with the right username and password, login fails.
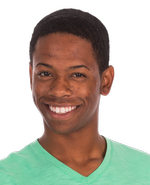
Ethan
So the code doesn't know whether it was the password that was wrong, or the username. Or even both.
Right! It doesn't need to know, since it wouldn't tell the user what was bad anyway. The whole login works, or the whole login doesn't.
OK, we're up to:
- If the username or password are missing:
- Return false (login failed)
- Hash the password
- Check the DB for a user record with the username and hashed password
- If it isn't there:
- Return false (login failed)
- UP TO HERE
- // If get to here, username and password are OK.
- Put user data into the session
- Return true (login OK)
Last bit:
- $userEntity = $stmnt->fetch();
- // Store data in session.
- $_SESSION['username'] = $userEntity['name'];
- $_SESSION['user_roles'] = explode(',', $userEntity['roles']);
- $_SESSION['logged_in'] = true;
- return true;
The user's data is in $userEntity
, the record fetched from the database.
Line 35 sets $_SESSION['logged_in']
. That's what isLoggedIn()
checks:
- /**
- * Is the current user logged in?
- * @return bool True if logged in, else false.
- */
- function isLoggedIn() {
- $loggedIn = false;
- if (isset($_SESSION['logged_in'])) {
- if ($_SESSION['logged_in']) {
- $loggedIn = true;
- }
- }
- return $loggedIn;
- }
$_SESSION['user_roles']
is used in hasRole($roleToCheck)
:
- /**
- * Does the current user have the given role?
- * @param string $roleName Role name, using consts above.
- * @return bool True if has role, else false.
- */
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
Remember, we want $_SESSION['user_roles']
to be an array, with an entry for each role the user has. That's an easy way to let users have more than one role. Then, we can ask whether the role we want to check ($roleName
) is in the array:
- return in_array($roleName, $_SESSION['user_roles']);
Here's what the debugger shows when checking for a role:
You can see that $_SESSION['user_roles']
is an array, with an element for each role the user has. There's just one element in the array here, but there could be more than one.
When we were making the users table, we decided to put the roles in a text field. If someone has more than one role, we'd put something like this in the roles field: admin,manager
. That is, we'd separate the role names with commas.
We want to make admin,manager
into the array, with the first item as admin
, and the second as manager
. How to do that? like this:
- $_SESSION['user_roles'] = explode(',', $userEntity['roles']);
explode(separator,string)
chops up a string, using the separator, and puts the pieces in array elements. For example,...
explode(' ', 'Doggos are great!')
... would return an array with three elements...
[0] => 'Doggos', [1] => 'are', [2] => 'great!'
If $userEntity['roles']
is 'admin,manager'
, then...
explode(',', $userEntity['roles'])
... would return an array with two elements...
[0] => 'admin', [1] => 'manager'
Logout
The functions isLoggedIn()
and hasRole($roleToCheck)
rely on session variables. For example:
- /**
- * Is the current user logged in?
- * @return bool True if logged in, else false.
- */
- function isLoggedIn() {
- $loggedIn = false;
- if (isset($_SESSION['logged_in'])) {
- if ($_SESSION['logged_in']) {
- $loggedIn = true;
- }
- }
- return $loggedIn;
- }
If $_SESSION['logged_in']
isn't there, isLoggedIn()
returns false. So does hasRole($roleToCheck)
.
So, an easy way to log someone out is to destroy the session. This is logout.php
, the whole thing:
- <?php
- require_once 'library/useful-stuff.php';
- logOut();
Here's logOut()
, from the library:
- /**
- * Logout.
- */
- function logOut() {
- session_destroy();
- header('Location: index.php');
- exit();
- }
session_destroy()
wipes out the session variables, all of them. That's all you need to do!
Exercise
Wombat user accounts
Earlier, you wrote an app to "list wombats:/exercise/wombat-list. Add user accounts to it, like the product app.
- Redirect non-logged in users to a login page.
- Add a form for username and password.
- Add
isLoggedIn()
andhasRole()
functions in your library. - A menu with a Logout link.
- A
users
table in your database. - Apply hashing to the password.
Submit the URL to your app, and a zip file of your code. Include a valid username and password for testing. The usual standards apply.