GET it
The simplest way to send data from a browser to the server is through a URL.
Here's a URL to a page on OU's Moodle instance:
- https://moodle.oakland.edu/course/view.php?id=242965
The URL has:
- The protocol,
https
- The server,
moodle.oakland.edu
- The page path,
/course/view.php
- And some strange stuff on the end,
?id=242965
The stuff on the end is a query string. The ?
signals the start of the string. There are parameters and value. So the parameter id
has the value 242965
.
You can pass more than one parameter, like this:
- something?name=Rosie&cuteness=12
An ampersand (&) separates the arguments. You can pass lots of them.
Who's the cutest?
Here's the code from last time:
- <?php
- $cutestDog = 'Rosie';
- $rating = 12;
- ?><!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <title>Cutest Dog</title>
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
- </head>
- <body>
- <h1>Cutest dog</h1>
- <p>The results are in!</p>
- <p>Cutest dog: <?php print $cutestDog; ?></p>
- <p>Rating: <?php print $rating; ?> out of 10.</p>
- </body>
- </html>
Let's change lines 2 and 3, to get data from the URL, like this:
- $cutestDog = $_GET['name'];
- $rating = $_GET['cuteness'];
So the program will be expecting two parameters in the URL: name
, and cuteness
.
Try this URL:
http://webappexamples.skilling.us/html/enter-php/cutest.php?name=Rosie&cuteness=12
In your browser, change the name and rating in the URL, and see what happens.
Try leaving out the data, that is, the ?
and everything after it.
Calculations
You can do calculation, just as in VBA. Here's another example:
- <?php
- $a = $_GET['a'];
- $b = $_GET['b'];
- ?><!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <title>Calculations</title>
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> </head>
- <body>
- <h1>Calculations</h1>
- <?php
- $aTimesB = $a * $b;
- print "<p>$a times $b is $aTimesB.</p>";
- $aDividedByB = $a / $b;
- print "<p>$a divided $b is $aDividedByB</p>";
- ?>
- </body>
- </html>
This one is expecting two GET parameters. It does some calculations, and shows the results.
Check out line 14:
- print "<p>$a times $b is $aTimesB.</p>";
If you use double quotes (") around a string, you can put variable names in the string, and PHP will substitute their values. This doesn't work with single quotes. So...
- print '<p>$a times $b is $aTimesB.</p>';
... would output exactly <p>$a times $b is $aTimesB.</p>
, with the dollar signs and variable names.
Sometimes you need to add braces, if PHP can't work out what you mean. For example, you could use...
- print '<p>{$a} times {$b} is {$aTimesB}.</p>';
The braces tell PHP that the thing between them should be replaced with a value. The braces themselves aren't output.
The code we've seen so far doesn't need braces, but you'll come across them later in the course.
OK, let's try it. Here's a URL:
http://webappexamples.skilling.us/html/enter-php/calculation.php?a=4&b=8
Try it.
Yay!
Here's one with the data missing.
http://webappexamples.skilling.us/html/enter-php/calculation.php
Try it.
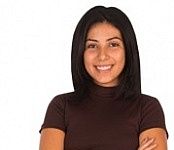
Adela
What's NAN mean?
Not a number.
Calculations can be as long as you want
PHP can do complex calculations, just like VBA. For example:
- $result = ($x + $y) * 4 - 27 / (90 + $z / 2.33);
() works the same as in VBA. Order of operations is as you expect.
What would this code output?
- $result = 2 + 3 * 4;
- print $result;
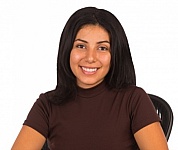
Adela
14. It would do the * first, then the +.
Right!
Another example
This one computes a triangle's hypotenuse from its sides.
- <?php
- // Compute the hypotenuse from the two sides.
- $side1 = $_GET['side'];
- $side2 = $_GET['other_side'];
- $hypotenuse = sqrt($side1 * $side1 + $side2 * $side2);
- ?><!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <title>Hypotenuse</title>
- </head>
- <body>
- <h1>Hypotenuse</h1>
- <p>Side 1: <?php print $side1; ?></p>
- <p>Side 2: <?php print $side2; ?></p>
- <p>Hypotenuse: <?php print $hypotenuse; ?></p>
- </body>
- </html>
It grabs two values from the end of the URL. They must called side
, and other_side
. Then it computes the hypotenuse, and outputs the results.
Video walkthrough
Here's a walkthrough, showing how to do an exercise. First, a sample exercise. It's fake. Don't try to submit it.
- - CUT SCREEN HERE - -
Work out how many grams of dilithium crystal it'll take to ship some quonks from Blutol to Skintal. You can try my solution.
- Each quonk takes 0.1 grams to ship (they're heavy).
- Add 0.3 grams of overhead for a shipment (launching to the Blutol orbital platform, loading onto a freighter, unloading at the Skintal orbital, and dropping to the planet).
- Add 0.4 grams for pirate insurance. It's optional.
Add input data to the end of the URL, like:
- .../shipping.php?quonks=311&insured=n
- .../shipping.php?quonks=9311&insured=Y
- .../shipping.php?quonks=666&insured=y
Notice that insured can be upper or lowercase. Assume both params are present, and have no errors.
- - CUT SCREEN HERE - -
Here's how I would do it.
Exercises
Circles
Write a PHP program to compute the circumference and area of a circle, from a radius in the URL. Make your output look like this program. Use 3.14159 as pi.
Submit your PHP file, and its URL on your server. To submit the file, attach it to your submission. Don't copy-and-paste its contents into the submission text area.
Tips
Write a program that takes meal_amount
from GET, and computes tips at different rates.
Here's some output:
Try it:
http://webappexamples.skilling.us/html/exercises/tips/tips.php?meal_amount=60
Use the rates shown in the screenshot. Submit the URL of your solution, and a zip of your PHP file.
Party planner
You run a dog party company. Write a program to estimate expenses, based on the number of dogs and pups who'll be invited.
dogs
and pups
are GET
input variables. You can try the program:
http://webappexamples.skilling.us/html/exercises/party-planner/party-planner.php?dogs=52&pups=38
Use 15% for the gratuity. Here are the costs per invitee:
Expense type | Per dog | Per pup |
---|---|---|
Food | 28 | 14 |
Drinks | 19 | 32 |
Games | 18 | 9 |
Gifts | 12 | 29 |
Clean up | 17 | 58 |
Submit the URL of your solution, and a zip of your PHP file.