Ivana's Boston
That add form...
Here's a form for inserting data from last time, after the user had tried to make a record, but validation has failed.
You know, that kinda looks like a form for editing data. There are fields. There's data in the fields. There's validation. Hmmm...
Hey y'all, what do we have to change to turn this...
... into a form for editing an existing comedian record?
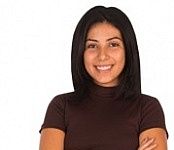
Adela
There's be data in the fields. Like, you'd say, "I want to edit comedian Joe Bloggs, and you'd see the form, but with Joe's data in the fields, instead of MTness.
Good! What else?
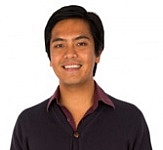
Ray
Well, the labels would be different. Add would be Edit.
Right, that would change.
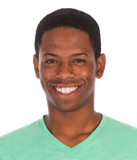
Ethan
When the user clicked Save, the processing program would so something different. Change data, rather than making a new record.
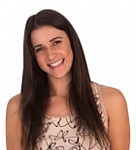
Georgina
Is that different SQL? We were using an INSERT, but that doesn't seem right.
Aye, that's true! There's an UPDATE statement. We'd have to use that.
Workflow
Here's the workflow diagram. Almost the same as before. I've added Sherlock Holmes where there are changes.
Workflow
The edit form program starts by loading the entity's existing data from the DB. The add page didn't load anything from the DB.
The form page still sends data to a save page, but it will be a different page from the insert one.
The processing page will run UPDATE, rather than INSERT.
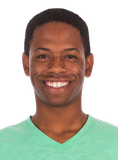
Ethan
Not many changes.
True.
The code
Here's the code for the edit form.
- <?php
- /**
- * Edit comedian.
- * This page validates, as well as showing the form.
- */
- session_start();
- require_once 'library/useful-stuff.php';
- $errorMessage = '';
- // Make variables for field values.
- // Initialize them.
- $comedianName = null;
- $comedianComments = null;
- // Get the id of the comedian to edit.
- $comedianId = getParamFromGet('comedian_id');
- $errorMessage = checkComedianId($comedianId);
- if ($errorMessage == '') {
- $comedianEntity = getComedianWithId($comedianId);
- if (is_null($comedianEntity)) {
- $errorMessage = "Sorry, problem loading comedian with id $comedianId.";
- }
- else {
- // Put the field values into variables.
- $comedianName = $comedianEntity['name'];
- $comedianComments = $comedianEntity['comments'];
- }
- }
- // Is there post data?
- if ($errorMessage == '' && $_POST) {
- // User filled in data and sent it to this page.
- // Grab the data sent.
- // It will overwrite existing data in the field variables.
- $comedianName = getParamFromPost('name');
- $comedianComments = getParamFromPost('comments');
- // Validate.
- $errorMessage .= checkName($comedianName);
- // Is everything OK?
- if ($errorMessage == '') {
- // No errors.
- // Stash data for processing later.
- $_SESSION['comedian_id'] = $comedianId;
- $_SESSION['comedian_name'] = $comedianName;
- $_SESSION['comedian_comments'] = $comedianComments;
- // Off to processing.
- header('Location: save-comedian.php');
- exit();
- }
- }
- ?><!doctype html>
- <html lang="en">
- <head>
- <?php
- $pageTitle = 'Edit comedian';
- require_once 'library/page-components/head.php';
- ?>
- </head>
- <body>
- <?php
- require_once 'library/page-components/top.php';
- print "<h1>$pageTitle</h1>\n";
- if ($errorMessage != '') {
- print "<p class='error-message'>$errorMessage</p>\n";
- }
- else {
- ?>
- <form method="post">
- <p>
- <label>Name:
- <input type="text" name="name" value="<?php
- if (! is_null($comedianName)) {
- print $comedianName;
- }
- ?>">
- </label>
- </p>
- <p>
- <label>Comments:
- <textarea name="comments"><?php
- if (! is_null($comedianComments)) {
- print $comedianComments;
- }
- ?></textarea>
- </label>
- </p>
- <p>
- <button type="submit">Save</button>
- </p>
- </form>
- <?php
- }
- require_once 'library/page-components/footer.php';
- ?>
- </body>
- </html>
Line 37 loads the existing data from the database. Lines 23 and 124 put the entity's field values into the variables $comedianName
and $comedianComments
.
Line 28 checks whether there is POST data. The first time the page is shown, there won't be. If there is post data, we put it into $comedianName
and $comedianComments
, replacing what was there from the record load.
If name passes validation, we stash data into the session for the save program (lines 40 to 42). Notice we stash the id as well. The add-comedian program didn't have a comedian id ready, since we were adding a new one. This program edits an existing record, so the id is there already.
The go-to-processing code (line 44) jumps to the different processing page. exit()
is needed, since we don't want to put anything else into the output.
Processing
Here's the code for processing.
- <?php
- session_start();
- require_once 'library/useful-stuff.php';
- // Get the data to save.
- $comedianId = $_SESSION['comedian_id'];
- $comedianName = $_SESSION['comedian_name'];
- $comedianComments = $_SESSION['comedian_comments'];
- // Save the data to the DB.
- $sql = "
- UPDATE comedians SET
- name = :name,
- comments = :comments
- WHERE
- comedian_id = :id;
- ";
- /** @var PDO $dbConnection */
- $stmnt = $dbConnection->prepare($sql);
- $stmnt->execute([
- 'id' => $comedianId,
- 'name' => $comedianName,
- 'comments' => $comedianComments
- ]);
- // Jump to the view page, passing new record id.
- header("Location: view-comedian.php?comedian_id=$comedianId");
Almost the same. We get the id for the record (line 5). We didn't have that for the add program.
An UPDATE instead of an INSERT (lines 9-15).
There used to be a line to get the last id that MySQL made, but we don't need that here.
The go-to-the-view-page code (line 24) is the same.
Operations links
Speaking of the view page, we could add some operation links to it:
Cool! Here's the HTML and CSS.
- "<p class='operations-container'>
- <a href='edit-comedian-form.php?comedian_id={$comedianId}'>Edit</a>
- <a href='create-comedian-form.php'>Add new</a>
- </p>\n";
- .operations-container a {
- margin-right: 2rem;
- }
What's next?
Add and edit are similar. Let's see if we can combine the add and edit programs into one program.