OK, so, you can type HTML into a file. Put the file on a server. When the server gets the corresponding URL, it send the HTML in the file to a browser.
But there's another way to make HTML. You can have a program write it. That's what the PHP language is for.
Who's the cutest?
Try this link:
It looks like:
Simple enough. <h1>
, and three <p>
s.
Let's check out the HTML source. Ctrl+U on Windows.
OK, nothing new.
Did you notice the URL?
Hmm, not .html
. It's .php
.
Let's go to the server, and check out the file on the server's disk drive:
- <?php
- $cutestDog = 'Rosie';
- $rating = 12;
- ?><!DOCTYPE html>
- <html lang="en">
- <head>
- <meta charset="UTF-8">
- <title>Cutest Dog</title>
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
- </head>
- <body>
- <h1>Cutest dog</h1>
- <p>The results are in!</p>
- <p>Cutest dog: <?php print $cutestDog; ?></p>
- <p>Rating: <?php print $rating; ?> out of 10.</p>
- </body>
- </html>
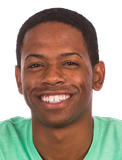
Ethan
Wait, that's not the HTML the browser got.
Right! The browser doesn't get this file directly. It gets the result of running this file on the server.
Lines 1-4, and lines 13 and 14, have PHP code. PHP is a language designed to be embedded in HTML.
Variables in PHP start with a $
. The line...
- $cutestDog = 'Rosie';
... puts the text Rosie
into the variable $cutestDog
.
Just as with any language, variables point to memory locations. $cutestDog
is a place in the computer's memory. You can put a value into it, and get a value out.
Now jump to line 13:
- <p>Cutest dog: <?php print $cutestDog; ?></p>
That outputs the value of $cutestDog
. Outputs where? At that point in the HTML.
Back to the top. The line...
- $rating = 12;
... puts 12 into the variable $rating
. The line...
- <p>Rating: <?php print $rating; ?> out of 10.</p>
... outputs $rating
in the HTML of the page.
Run the program, and you get HTML to send to the browser.
It's on the server
PHP runs on the server. The user's browser just gets HTML. It doesn't know where the HTML came from. It just renders what it gets.
When XAMPP installed Apache, it also installed a PHP interpreter, a program that can run PHP programs. XAMPP hooked up the PHP interpreter to Apache.
So:
- The browser sends a URL ending in PHP to the web server (Apache).
- Apache grabs the file matching to URL from the file system (disk drive).
- Apache notices the
.php
extension. It sends to file to the PHP interpreter. - The interpreter runs the code.
- The interpreter sends the resulting HTML to Apache.
- Apache send the HTML to the client (the browser).
Renata is cute, too
Let's change the program. You can try the new one
- <?php
- $cutestDog = 'Renata';
- $rating = 10;
- ?><!DOCTYPE html>
- ...
- <p>The results are in!</p>
- <p>Cutest dog: <?php print $cutestDog; ?></p>
- <p>Rating: <?php print $rating; ?> out of 10.</p>
- ...
Only the marked code is changed. Here's the HTML the browser got.
Lines 10 and 11 have changed. There's no PHP, just the results of running the PHP.
Syntax
The syntax is different from VBA. Here's the PHP again:
- <?php
- $cutestDog = 'Renata';
- $rating = 10;
- ?><!DOCTYPE html>
- ...
- <p>The results are in!</p>
- <p>Cutest dog: <?php print $cutestDog; ?></p>
- <p>Rating: <?php print $rating; ?> out of 10.</p>
- ...
PHP is between <?php
and ?>
. This is so the PHP interpreter knows what to pay attention to.
Variables start with $
, as I said. Variables don't need to be declared, that is, there is no Dim
statement, as in VBA.
Remember that in VBA, you give variables a type, usually Single
or String
. PHP lets variables be any type they want. Type depends on how the variable is used, not how it is declared. You'll see this as we go.
Each statement in PHP must end with a semicolon (;). That's true of many languages.
Naming standards
We'll use some coding standards in the course.
Use camel case for variable names. All characters lowercase, except when you have a multi-word name. Make the first character of the second and subsequent words uppercase. Examples:
- $dogCount
- $averageAcidity
- $monthlyPayment
Check the coding standards page for all the deets.
Up next
How can we get the dog name and cuteness from the user?