So far, we've seen two widget types: text, and textarea. There are others, though. This lesson is about checkboxes...
...and options, also called radio buttons...
Category
They need some special handling.
Checkbox
Here's a checkbox widget:
There are two parts to it:
- The small box you check
- The label
The box and the label are linked. Click on the label, and the box changes. Try it.
Here's the HTML:
- <label>
- <input type='checkbox' name='sponsored' checked>
- Sponsored
- </label>
The input
and the label text are wrapped in the label
tag. That's what ties them, so that clicking on the label toggles the box.
The name
attribute is the name of the widget's value in the $_POST array.
- Form page:
- <input type='checkbox' name='sponsored' checked>
- Processing page:
- $sponsored = $_POST['sponsored'];
name
works the same on every widget.
checked
tells you the value of the widget. If checked
is present in the input
tag...
- <input type='checkbox' name='sponsored' checked >
... the box is checked. If it's not there...
- <input type='checkbox' name='sponsored'>
... the box is not checked.
So, how does the processing page tell whether the user checked the box? If they have, there'll be a value for the widget in the $_POST
array, and it will be on
.
- if ( isset($_POST['sponsored']) && $_POST['sponsored'] == 'on' ) {
- // The user checked the box.
- ...
- }
- else {
- // The user did not check the box.
- ...
- }
We'll see how this works later.
Option (radio) buttons
Checkboxes are for values that are on, or off. Options buttons, also called radio buttons, apply when you have a field with several different values. Here's an example:
Category
Category is one of core, lifestyle, or academic. Click one, and the others turn off.
Each option has its own input
tag, and label. For example:
- <label>
- <input type="radio" name="category" value="c">
- Core</label>
Here's another:
- <label>
- <input type="radio" name="category" value="l">
- Life style</label>
Notice they have the same name
. That's what makes them different options for the same value. There can be as many <input type="radio" name="category"
as you like. Click on one of them, and all other radio
s with the same name turn off. Only one radio
with the name category
can be checked.
$_POST['category']
will have the contents of the value
property of the radio
that's selected.
If the user clicked Life style
above, what value would the $_POST['category']
have?
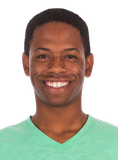
Ethan
The one with the label Life style has value="l"
, so it would be l.
Correct!
A club editing form
Here are the fields in a clubs
table:
Id, name, and comments are familiar. category
is a one-character field, that's either c (core), l (life style), or a (academic). There are three different values, so we'll use option buttons for that.
sponsored
is a one-character field, that's either y or n. A good use for a checkbox.
Let's make an edit form that looks like this:
Here's the code that loads one club's data from the DB:
- $sql = 'select * from clubs where club_id = :id;';
- /** @var PDO $dbConnection */
- $stmnt = $dbConnection->prepare($sql);
- $stmnt->execute([':id' => $clubId]);
- if ($stmnt->rowCount() == 0) {
- $errorMessage = "Sorry, couldn't find a club with an id of $clubId";
- }
- else {
- // Put the field values into variables.
- $clubRow = $stmnt->fetch();
- $clubName = $clubRow['name'];
- $clubCategory = $clubRow['category'];
- $clubSponsored = $clubRow['sponsored'];
- $clubComments = $clubRow['comments'];
- }
$clubCategory
will be a single letter, c, l, or a. $clubSponsored
will be y or n. Here's what the debugger shows, after loading a club's record:
The category widgets
Here's the PHP to show the categories widgets. This code would go into an add/edit form for clubs.
- <p>
- Category<br>
- <label>
- <input type="radio" name="category" value="c" <?php
- if ($clubCategory == 'c') {
- print "checked";
- }
- ?> >
- Core</label><br>
- <label>
- <input type="radio" name="category" value="l" <?php
- if ($clubCategory == 'l') {
- print "checked";
- }
- ?> >
- Lifestyle</label><br>
- <label>
- <input type="radio" name="category" value="a" <?php
- if ($clubCategory == 'a') {
- print "checked";
- }
- ?> >
- Academic</label>
- </p>
There's an <input type="radio" name="category"
for every value, and an if
to output checked
is category
has that value.
The sponsored widget
Here's the code for a sponsored widget, that would go into an add/edit form for a club.
- <label>
- <input type='checkbox' name='sponsored' <?php
- if ($clubSponsored == 'y') {
- print 'checked';
- }
- ?> >
- Sponsored
- </label>
Processing
On an add/edit page, there's PHP to send the fields' values for processing. Here's what we'd have for clubs.
- // Is everything OK?
- if ($errorMessage == '') {
- // No errors.
- // Stash data for processing later.
- $_SESSION['club_id'] = $clubId;
- $_SESSION['club_name'] = $clubName;
- $_SESSION['club_category'] = $clubCategory;
- $_SESSION['club_sponsored'] = $clubSponsored;
- $_SESSION['club_comments'] = $clubComments;
- // Off to processing.
- header('Location: save-club.php');
- exit();
- }
What about the processing page itself? There'd be extra stuff in the code that takes the sponsored widget's value from POST
.
Checkboxes send on
into POST
when the box is checked. Otherwise, they send nothing. We need to convert the on-or-nothing value in POST
to the y-or-n we'll put into the database.
- // Is there post data?
- if ($errorMessage == '' && $_POST) {
- // User filled in data and sent it to this page.
- // Grab the data sent.
- // It will overwrite existing data in the field variables.
- $clubName = getParamFromPost('name');
- $clubCategory = getParamFromPost('category');
- $clubSponsored = getParamFromPost('sponsored'); Returns "on" or null.
- // Convert checkbox widget to stored field value.
- if ($clubSponsored == 'on') {
- $clubSponsored = 'y';
- }
- else {
- $clubSponsored = 'n';
- }
- $clubComments = getParamFromPost('comments');
- // Validate.
- $errorMessage .= checkName($clubName);
- $errorMessage .= checkClubCategory($clubCategory);
- $errorMessage .= checkClubSponsored($clubSponsored);
You can see how that works in lines 10-15. on
becomes y
. Everything else (including the null
that getParamFromPost()
returns when it can't find a value) becomes n
.
Up next
One more widget to go: the dropdown select thing.