Here's a task:
- - CUT SCREEN HERE - -
Write a program telling how many peanuts to get, depending on an animal type. You can try it for a goat: http://webappexamples.skilling.us/html/no-not-there/no-not-there.php?animal=goat
Here's some sample output, for ?animal=goat
at the end of the URL:
All input is in lowercase.
Here are the messages for different animals:
Animal | Message |
---|---|
Squirrel | Get a few peanuts. |
Goat | Get a lot of peanuts. |
Elephant | Get lots and lots and lots of peanuts! |
Other | I don't know how many peanuts you'll need. |
- - CUT SCREEN HERE - -
Let's watch Ray work through it.
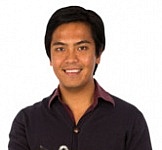
Ray
OK, looks easy enough. I'll start by checking the example in the last lesson.
GET... if
, ==
... OK.
So, start by GETting input. The field is called animal, so...
- <?php
- // Get input.
- $animal = $_GET['animal'];
Hmm... the output wants to show the animal.
Looks like a p
tag. Let me check. I'll look at the sample page, then check the HTML on it:
That will show me the HTML created by the program.
I'll find the HTML showing the animal:
Right, it's a p
tag. So...
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
Is that right?
Is the code right so far?
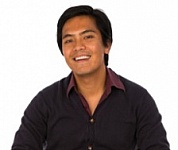
Ray
Now for the if
. Start with squirrels. Let me check the output message:
Animal | Message |
---|---|
Squirrel | Get a few peanuts. |
Goat | Get a lot of peanuts. |
Elephant | Get lots and lots and lots of peanuts! |
Other | I don't know how many peanuts you'll need. |
So that would be...
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
- // Print right message.
- if ($animal == 'squirrel') {
- print '<p>Get a few peanuts.</p>';
- }
OK, now the others...
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
- // Print right message.
- if ($animal == 'squirrel') {
- print '<p>Get a few peanuts.</p>';
- }
- elseif ($animal == 'goat') {
- print '<p>Get a lot of peanuts.</p>';
- }
- elseif ($animal == 'elephant') {
- print '<p>Get lots and lots and lots of peanuts!</p>';
- }
- else {
- print "<p>I don't know how many peanuts you'll need.</p>";
- }
Alright. Just need to copy the page template from that earlier lesson, paste it in, change the title and heading, and there it is!
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
- // Print right message.
- if ($animal == 'squirrel') {
- print '<p>Get a few peanuts.</p>';
- }
- elseif ($animal == 'goat') {
- print '<p>Get a lot of peanuts.</p>';
- }
- elseif ($animal == 'elephant') {
- print '<p>Get lots and lots and lots of peanuts!</p>';
- }
- else {
- print "<p>I don't know how many peanuts you'll need.</p>";
- }
- ?><!doctype html>
- <html lang="en">
- <head>
- <title>Peanuts needed</title>
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
- <link rel="stylesheet" href="styles.css">
- </head>
- <body>
- <h1>Peanuts needed</h1>
- </body>
- </html>
Time to test it!
Will the code work? If not, what would it show?
Here's what you get: http://webappexamples.skilling.us/html/no-not-there/no-not-there-wrong1.php?animal=goat
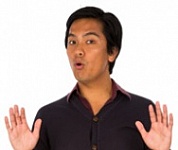
Ray
Wait, that's not it! What's going on?
I'll right-click and check the HTML my program made...
Oh, man! I want the animal and peanuts HTML inside the body
, like this:
Here's Ray's code:
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
- // Print right message.
- if ($animal == 'squirrel') {
- print '<p>Get a few peanuts.</p>';
- }
- elseif ($animal == 'goat') {
- print '<p>Get a lot of peanuts.</p>';
- }
- elseif ($animal == 'elephant') {
- print '<p>Get lots and lots and lots of peanuts!</p>';
- }
- else {
- print "<p>I don't know how many peanuts you'll need.</p>";
- }
- ?><!doctype html>
- <html lang="en">
- <head>
- <title>Peanuts needed</title>
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
- <link rel="stylesheet" href="styles.css">
- </head>
- <body>
- <h1>Peanuts needed</h1>
- </body>
- </html>
What should Ray do to fix the code?
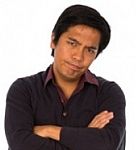
Ray
What am I going to do?
Well, like Kieran says, when in doubt, find an example.
Indeed!
He's right. I do say that.
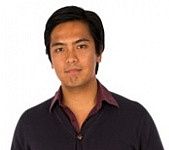
Ray
Let's see... here's some code from the last lesson:
- <?php
- $dogs = $_GET['dogs'];
- // Compute message, and CSS class.
- $message = '';
- $class = '';
- if ($dogs < 1) {
- $message = "You don't have doggos! Boo! Get doggos!";
- $class = 'argh';
- }
- ...
- <!DOCTYPE html>
- <html lang="en">
- <body>
- <h1>Dogs</h1>
- <p>Do you have enough dogs?</p>
- <p>Number of dogs: <?= $dogs ?></p>
- <p class="<?= $class ?>"><?= $message ?></p>
- ...
Oh, I see it! I print stuff right away:
- <?php
- // Get input.
- $animal = $_GET['animal'];
- print "<p>Your animal: $animal </p>";
- ...
- <!DOCTYPE html>
- <html lang="en">
- <body>
But the example doesn't. It prints a variable later:
- <?php
- $dogs = $_GET['dogs'];
- ...
- ?><!DOCTYPE html>
- ...
- <body>
- <h1>Dogs</h1>
- <p>Do you have enough dogs?</p>
- <p>Number of dogs: <?= $dogs ?></p>
The same in the if
. I print it immediately:
- if ($animal == 'squirrel') {
- print '<p>Get a few peanuts.</p>';
- }
- ...
- <!DOCTYPE html>
- <html lang="en">
- <body>
The example puts the output it wants into variables, and prints them later:
- $message = '';
- $class = '';
- if ($dogs < 1) {
- $message = "You don't have doggos! Boo! Get doggos!";
- $class = 'argh';
- }
- ...
- <!DOCTYPE html>
- <html lang="en">
- <body>
- ...
- <p class="<?= $class ?>"><?= $message ?></p>
- ...
Get the order right
You need to output the HTML in the right order, so you make a valid HTML page. Often. that means:
- The top of your code puts the output you want into variables.
- The bottom of your code outputs the variables.
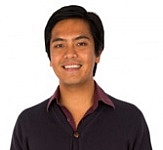
Ray
OK, I think I've got it now. I'll use variables.
- <?php
- // Set up var to track results.
- $peanutsNeeded = '';
- // Get input.
- $animal = $_GET['animal'];
- // Adjust peanuts needed.
- if ($animal == 'squirrel') {
- $peanutsNeeded = 'Get a few peanuts.';
- }
- elseif ($animal == 'goat') {
- $peanutsNeeded = 'Get a lot of peanuts.';
- }
- elseif ($animal == 'elephant') {
- $peanutsNeeded = 'Get lots and lots and lots of peanuts!';
- }
- else {
- $peanutsNeeded = "I don't know how many peanuts you'll need.";
- }
- ?><!doctype html>
- <html lang="en">
- <head>
- <title>Peanuts needed</title>
- <meta charset="utf-8">
- <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
- <link rel="stylesheet" href="styles.css">
- </head>
- <body>
- <h1>Peanuts needed</h1>
- <p>Your animal: <?= $animal ?></p>
- <p><?= $peanutsNeeded ?></p>
- </body>
- </html>
You can try it: ": http://webappexamples.skilling.us/html/no-not-there/no-not-there.php?animal=goat
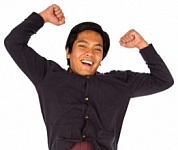
Ray
Weehoo! I rock!
When you think about it, you're writing code (in PHP), that writes code (in HTML). Kinda mind blowing.
You have to write your PHP so that it writes the correct HTML. Using variables helps you do that.