So far, we've put log files in the same folders as the webpages that write to them.
Webpages and log files are in the same folders. What security issues might that cause?
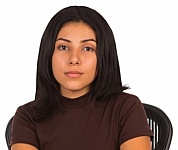
Adela
Oh. People might be able to see the log files, by typing in their URLs.
Right! Not good.
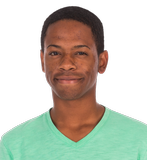
Ethan
But they'd have to know the names of the log files, to put them in the URLs. How would they know that?
There are URL guessing programs. They try lots of combinations, until they get lucky.
If the software is open source, hackers will have the program code. They can check it, to see what log files the programs make.
Even if the software is from a well-known vendor, like SAP, then many companies will be using it. Anyone who works with the software might know what the log file names are.
Another principle:
Security by obscurity doesn't work
Keep your code secret, and nobody can hack it, right? Well... it's not that easy.
Someone will figure out. And when they do, you are vulnerable.
Move the log files
To understand the solution, remember how web servers work. The server has a disk drive. One folder on the drive is the root of the website.
Files in this folder (or its subfolders) are accessible over the web.
Files that aren't in this folder are not accessible over the web.
PHP programs run on the server, and can access the server's entire file system. So, our PHP program could write log files in a folder that isn't under the web root. Problem solved!
file_put_contents
We can tell file_put_contents()
to put data anywhere. Say I made a folder on my PC called D:\logfiles
. I could use that in file_put_contents()
:
- $filePath = 'D:/logfiles/dog-log.txt';
- $logEntry = "Cutest dog: $cutestDog Rating: $rating\n";
- file_put_contents($filePath, $logEntry, FILE_APPEND);
- ?><!DOCTYPE html>
(Notice that I used / rather than \ in the path. In PHP, / works on Windows, Mac, and Linux, so let's use that.)
Does it work?
Yes! There's the file! W00t!
D:\logfiles
isn't under the web root, so the log file isn't accessible through a URL. Hackers can try to guess the log file's URL as much as they want. The file doesn't have a URL. So... good luck with that, hackers!
Job interview
"I learned how to protect audit logs from URL guessing attacks" sounds good in a job interview.
Moving to your web server
This...
- $filePath = 'D:/logfiles/dog-log.txt';
- $logEntry = "Cutest dog: $cutestDog Rating: $rating\n";
- file_put_contents($filePath, $logEntry, FILE_APPEND);
- ?><!DOCTYPE html>
... works on my PC, but won't work on my Reclaim server. Linux doesn't use drive letters, for a start. So, I need to change the path to the log files to something that will work on Linux.
Here's ye olde cPanel file manager:
The is where my files go on the server. This is not a URL, but a file path.
I can make a folder for my log files.
There it is, next to an existing folder called logs
.
Look in the new folder, and it's MT.
So, I made new folder, at /home/cullenma/logfiles
.
Let's use that in some PHP.
- <?php
- // Get data from the URL.
- $cutestDog = $_GET['name'];
- $rating = $_GET['cuteness'];
- // Log.
- $filePath = '/home/cullenma/logfiles/dog-log.txt';
- if ( file_exists($filePath) && filesize($filePath) > 10000) {
- header('Location: log-file-size-warning.php');
- exit();
- }
- $logEntry = "Cutest dog: $cutestDog Rating: $rating\n";
- file_put_contents($filePath, $logEntry, FILE_APPEND);
- ?><!DOCTYPE html>
- ...
You can see the path to the log file in line 6.
OK, I go to this page, then look in the cPanel file manager again. Reload, and see:
W00t! Now I can write logs on my Reclaim server, and the log files aren't accessible over the web. The log files are limited in size, too. Take that, hackers! Sucks to be you!
Up next
Let's talk about what data will be in each event log record, and how we'll format it.