Checking roles
Let's write code for the user list program. Remember, the user list should only be visible to admins. Anyone else who tries to access the page should get an error.
This code will do it.
- <?php
- // List users.
- require_once 'library/useful-stuff.php';
- $errorMessage = '';
- // Check permission.
- if (! hasRole(ROLE_NAME_ADMIN)) {
- header('HTTP/1.0 403 Forbidden');
- exit();
- }
- $userEntities = getAllUsers();
Line 3 adds our code library, as usual. Line 6 does the check:
- if (! hasRole(ROLE_NAME_ADMIN)) {
In the code library, we'll write a function called hasRole()
. It takes one parameter: the role to check for. hasRole()
returns true if the current user has that role, or false if not.
So, if the current user does not have the admin role, then:
- header('HTTP/1.0 403 Forbidden');
- exit();
We used header()
before to redirect the browser to another page. This time, we tell the browser that it's forbidden from accessing the page. The exit()
is necessary, since header()
only works when it's the first thing on the page, and nothing follows it.
We also want to restrict access to the sales page, to managers. Here's code from that page.
- // Check permission.
- if (! hasRole(ROLE_NAME_MANAGER)) {
- header('HTTP/1.0 403 Forbidden');
- exit();
- }
This checks a different role, manager instead of admin.
For this code to work, we need to write hasRole()
. So, how is hasRole()
going to know whether the current user is logged in?
Sessions
We're going to use sessions to keep track of whether people are logged in. We used sessions earlier. Let's remind ourselves.
Say there are four people using your app.
Each person will have a separate chunk of memory on the server, accessed with $_SESSION
. We can keep any data we want there. $_SESSION
for Sarah is different from $_SESSION
for Linda is different from $_SESSION
for Teagan is different from $_SESSION
for Joe. Put data into Sarah's $_SESSION
, and the others will not be affected.
Suppose Linda and Sarah are logged in, but the others are not. We can put data in their $_SESSION
s, to show:
- They're logged in
- Their username
- Their roles
Writing hasRole()
hasRole()
takes one param: a permission to check. It returns true if the current user has the role, false if not.
Here's code for it:
- session_start();
- ...
- const ROLE_NAME_ADMIN = 'admin';
- const ROLE_NAME_MANAGER = 'manager';
- ...
- /**
- * Is the current user logged in?
- * @return bool True if logged in, else false.
- */
- function isLoggedIn() {
- $loggedIn = false;
- if (isset($_SESSION['logged_in'])) {
- if ($_SESSION['logged_in']) {
- $loggedIn = true;
- }
- }
- return $loggedIn;
- }
- /**
- * Does the current user have the given role?
- * @param string $roleName Role name, using consts above.
- * @return bool True if has role, else false.
- */
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
Check out lines 3 and 4:
- const ROLE_NAME_ADMIN = 'admin';
- const ROLE_NAME_MANAGER = 'manager';
const
defines a constant. When PHP finds ROLE_NAME_ADMIN
, it substitutes the string admin
. When it finds ROLE_NAME_MANAGER
, it substitutes manager
.
This lets us write code like:
- if (! hasRole(ROLE_NAME_MANAGER)) {
It's easy to read, and hard to mess up. Without the constant, we might write...
- if (! hasRole('manager')) {
... in one place, and...
- if (! hasRole('manager ')) {
... in another. One would work, the other not, because of the extra space. That can be hard to debug.
Here's hasRole()
:
- /**
- * Does the current user have the given role?
- * @param string $roleName Role name, using consts above.
- * @return bool True if has role, else false.
- */
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
First, if the current user isn't logged in, they don't have any roles, and hasRole()
should return false. That's what lines 26 to 28 do.
Line 29 uses PHP's built-in in_array()
function. You give it a value you want to search for, and the array you want to search. So, for this to work, we need an array in the session, with all of the user's roles in it.
Here's some examples of how in_array()
works.
$needle |
$haystack |
in_array($needle, $haystack) |
---|---|---|
dog | [llama, cat, dog] | true |
dog | [dog, llama, cat] | true |
camel | [llama, cat, dog] | false |
dog | [dog] | true |
Here's what the debugger shows, on the first line of hasRole()
, when checking for the admin role:
$roleName
is admin
in this case. You can see that $_SESSION['user_roles']
is an array. It has one element for each role the user has. This allow a user to have multiple roles.
So, for...
- in_array($roleName, $_SESSION['user_roles'])
... $roleName
is admin
, and $_SESSION['user_roles']
is ['admin']
. admin
is in the array, so in_array()
is true. The code...
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
... returns the value of in_array()
.
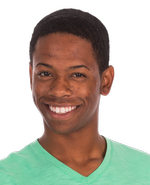
Ethan
OK, I get that, but it will only work if $_SESSION['user_roles']
has the current user's roles. Is that something PHP does for us automatically?
Good question. No, it isn't automatic. The login program will look up the user's roles in a database, and put them in $_SESSION['user_roles']
. We'll look at that later.
Right now, we're looking at how checks are made, so we know what the login program has to do.
isLoggedIn()
hasRole()
starts by calling isLoggedIn()
:
- function hasRole(string $roleName) {
- if (!isLoggedIn()) {
- return false;
- }
- return in_array($roleName, $_SESSION['user_roles']);
- }
Let's write that.
- /**
- * Is the current user logged in?
- * @return bool True if logged in, else false.
- */
- function isLoggedIn() {
- $loggedIn = false;
- if (isset($_SESSION['logged_in'])) {
- if ($_SESSION['logged_in']) {
- $loggedIn = true;
- }
- }
- return $loggedIn;
- }
We'll make the login program set $_SESSION['logged_in']
to true
if the user is logged in.
The code uses the flag pattern. Set the flag to false to start (line 11). Then, if $_SESSION['logged_in']
exists (line 12), and it's true (line 13), set the flag to true. Return the flag (line 17).
Use a variable as a flag. Set the flag if any of a number of things happens. After, check the flag to see if any of those things happened.
What login should do
This is what we'll want the login program to do, when we write it:
- For a valid login, put
true
in$_SESSION['logged_in']
- For a valid login, put the user's roles in
$_SESSION['user_roles']
Then hasRole()
and isLoggedIn()
will work.
Up next
Let's adjust the top menu depending on the user's roles.