Log in
If you're a student, please log in, so you can get the most from this page.
Not graded. So why do it?
Here's code that does the same thing: conditionally sets a variable. Compare the code, and you'll see the difference.
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Write a program that gets an animal's height from the URL. It outputs "That's a tall animal!" if the height is more than 2 (meters). Otherwise it outputs "That's not a tall animal."
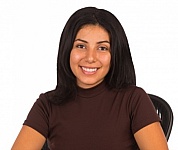
Adela
Here's what I wrote.
- <?php
- $height = $_GET['height'];
- if ($height > 2) {
- $message = "That's a tall animal!";
- }
- else {
- $message = "That's not a tall animal.";
- }
- print "<p>$message</p>\n";
Nice! Good work!
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Not graded. So why do it?
Explain this code. How would you improve it?
- <?php
- $pieType = $_GET['pie'];
- $numberPiesToBuy = $_GET['number'];
- if ($pieType == 'pecan' || $pieType == 'custard') {
- $numberPiesToBuy += 1;
- }
- if ($numberPiesToBuy == 1) {
- $noun = 'pie';
- }
- else {
- $noun = 'pies';
- }
- print "<p>Buy $numberPiesToBuy $noun.</p>";
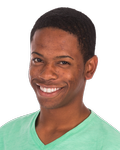
Ethan
The first two lines get input from the URL: pie type, and number.
If it's a pecan or custard, add another one.
Output how many pies to buy. Use "pie" if it's one pie, and "pies" if there's more than one.
I'd improve it by trimming the text, and making it lowercase.
Nice! Good job!
Explain what this code does.
- <?php
- $order = $_GET['order_amount'];
- $primeMember = $_GET['prime'];
- if ($order > 25 || $primeMember == 'yes') {
- $shipping = 0;
- }
- else {
- $shipping = $order / 10;
- }
- $orderTotal = $order + $shipping;
- print "<p>Order: $order<br>Shipping: $shipping<br>Total: $orderTotal</p>";
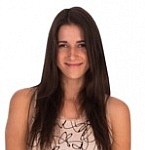
Georgina
It works out shipping costs for an order. It's free for Prime members, or if the order is for more than $25.
Got it! Thanks!
Not graded. So why do it?
Explain what this code does.
- <?php
- $animal = $_GET['animal'];
- if ($animal == 'giraffe') {
- $faveGame = 'Basketball';
- }
- else if ($animal == 'lion') {
- $faveGame = 'Tag';
- }
- else if ($animal == 'tiger') {
- $faveGame = 'Operation';
- }
- else if ($animal == 'penguin') {
- $faveGame = "Don't Break the Ice";
- }
- else {
- $faveGame = '(Unknown)';
- }
- print "<p>A {$animal}'s fave game is $faveGame.</p>";
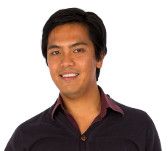
Ray
It tells you what an animal's favorite game is. It only knows a few animals. It says unknown if it doesn't know the animal.
Good! That's what it does.
Explain what this code does.
- <?php
- $team1Score = $_GET['team1'];
- $team2Score = $_GET['team2'];
- $spread = abs($team1Score - $team2Score);
- if ($spread == 0) {
- $comment = 'A tie!';
- }
- else if ($spread < 3) {
- $comment = 'A close game';
- }
- else if ($spread < 8) {
- $comment = 'A comfortable win';
- }
- else {
- $comment = 'A thrashing!';
- }
- print "<p>$comment</p>";
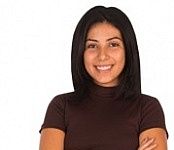
Adela
It outputs a different comment, depending how much one team beat the other.
Got it!