In the goats app, several pages load goat data, given a goat id. Rather than write the code several times, I wrote it once, and made a function for it:
- /**
- * Get goat record for given id.
- * @param int $goatId Id to look up.
- * @return array|null Record from DB, or null is not found, or bad id.
- */
- function getGoatWithId($goatId) {
- global $dbConnection;
- $result = null;
- if (! is_null($goatId) && is_numeric($goatId)) {
- $sql = "select * from goats where goats.goat_id = :goat_id_to_show;";
- /** @var PDO $dbConnection */
- $stmnt = $dbConnection->prepare($sql);
- $isQueryWorked = $stmnt->execute([':goat_id_to_show' => $goatId]);
- if ($isQueryWorked && $stmnt->rowCount() == 1) {
- $result = $stmnt->fetch();
- }
- }
- return $result;
- }
I put this in a code library, that's included on all pages, ready to be used as needed.
The first few lines document the function. Everyone knows what the function should do, and PHPStorm can show help for it.
What are the advantages of reusing code like this?
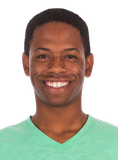
Ethan
Well, you save time, writing it once.
Yes. Time is money, especially in programming, since it's all intellectual work.
Anything else?
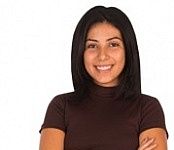
Adela
How about reliability? You only have one piece of code to test.
Right! Good thinking.
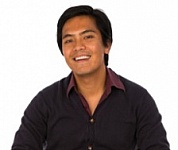
Ray
Yeah, but if it had a problem, it would be everywhere right?
Yes! That's right. Good thinking! Still, it's better to know where an error is, and fix it once, rather than not be sure about errors.
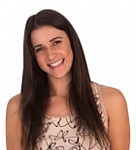
Georgina
Oh, and changes, too. If you need to change something, you only change it once.
Aye!
Bottom line: reuse is good.